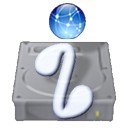
Writing i-Package scripts
Scripts may be written in any language. They may even be executables, though this is not recommended because this prevents the user from inspecting them and that is a security risk. Scripts get the following environment variables from i-Installer when running:
- II2LOGLEVEL
- See preferences
- II2USER
- The real user that is running i-Installer.
- II2AUTHENTICATED
- If the script is running authenticated.
- II2PKGSTAMP
- The time in seconds since 1970 when this i-Package was last modified by its author
- II2PKGSTAMPDISPLAY
- A user-friendly format for the previous variable
- II2BUILDSTAMP
- The time in seconds since 1970 when this version of i-Installer was compiled
- II2BUILDSTAMPDISPLAY
- A user-friendly format for the previous variable
- II2PKGDIR
- The directory that is the i-package file bundle (ending on ".ii2")
- II2INSTALLDIR
- The install location. See preferences
- II2PKGURL
- The URL of this package where rmeote updates etc. can be found
- II2RESOURCES
- The Resources directory of i-Installer, where support programs can be found. The following support programs are available: gnutar, gzcat, bzcat, gpg, basename, dirname, env, file, hexdump, openssl. Most of these are programs that are available on any Mac OS X system, but since Mac OS X offers the option not to install the BSD subsystem they might not be available on every Mac OS X installation and i-Installer therefore needs to bring its own copy.
- II2DOSERVER (optional)
- The name of the Distributed Objects server for interaction between script and i-Installer for this particular script while it is running. Optional. Depends on the interactive flag being set in the package.
- II2SELECTEDSETS
- Which sets have been selected. Otional. Depends on the availability of sets in the package.
Furthermore, using the doalerter tool, it is possible to communicate settings to i-Installer.
Scripts may interact with i-Installer to provide the following functionality:
- An informational alert sheet on the package window
- An alert sheet containing a PopUp button
- An alert sheet containing a List to select items from
- An alert sheet containing a List of checkboxes to be turned off or on
- An alert sheet containing an input field to enter data
- A command to try to open another i-Package through an URL.
The workhorse for this is a program that lives inside i-Installer's Resources, called doalerter (Distributed Objects alerter). doalerter has the following options (this information might be out of date, new features that have not been released into this help information can be seen by inspecting the source code for doalerter):
- -s doserver
- Required. doserver is the name of the Distributed Objects server to talk to. Normally, this means using the II2DOSERVER environment variable.
- -t type
- Required. Type of interaction. type needs to be one of Alert, Select (List), Choice (PopUp), Table (List of checkboxes), Input, Open, Environment SetSelectorSets, GetSelectorSets. SetConfiguredSelectorSets, UnsetConfiguredSelectorSets, SetInstalledSelectorSets, UnsetInstalledSelectorSets. All are alert sheets of a sort, except Open, Environment and the SelectorSet modes.
- -T title
- title is the Title of the alert sheet.
- -I info
- info is the informational message of the alert sheet.
- -E variablesetting
- variablesetting is in the form "FOO=bar" and implies that variable FOO is set to the value bar.
- -C choices
- choices is the choice array if applicable (Select, Choice, Table). This is a string made o strings separated with the separation character. The first character of each item decides if the item is selected at startup of the sheet. A + sign means selected, a - sign means deselected (if applicable: Select, Table)
- -S sepchar
- sepchar is the separation character inside the choice string, by default this is the bar character |.
- -i initialvalue
- initialvalue is the initial value of the sheet, if applicable (Input, Choice).
- -1 okbutton
- okbutton is the text on the OK button. If not given, the value is "OK".
- -2 otherbutton
- otherbutton is the text on the Other button. If not given, the button is not available.
- -3 cancelbutton
- cancelbutton is the text on the Cancel button. If not given, the button is not available.
- -v
- Verbose report of progress
- -V
- Verbose report of result. When -V is given, Alert will report the text of the button pressed instead of the numerical value, Choice will report the text of the item selected and not the index, Select and Table will report textual instead of numerical (index) output. Input is not influenced.
- -u url
- url is the url to open if applicable (Open)
Result is reported on standard output.
A special consideration for remove scripts
At the end of a succesful (exit value is 0) run of a remove script. i-Installer will try to remove the time stamp file that was installed at the install location to mark the software installation. But scripts may return a success value without having removed the software. For instance, the TeX remove script is shared and you can select to remove nothing and it will succesfully finish.
For this situation, a special mechanism is available starting from i-Installer 2.20.0. When for the package foo.ii2 the special file .foo.ii2.K is available, it will be removed instead of the real time stamp. Thus, when the remove script in the end has not removed the installation, it can create that file to make sure the install time stamp survives.
Example
For more examples, I advise to look at the configuration script of existing packages. Note that this example could be improved upon. Instead of giving a hard coded URL, it could have been adapted from the II2PKGURL variable, but that is left as an excercise for the reader.
#!/bin/sh
if [ ! -x "${II2RESOURCES}/basename" ]
then
echo "### This package needs a more recent version of i-Installer. Bailing out...\n" >&2
exit 1
fi
PROGNAME=`"${II2RESOURCES}/basename" "$0"`
echo "### ${PROGNAME}: Started..."
if [ "${II2INSTALLDIR}" == "" ]
then
echo "### ${PROGNAME}: II2INSTALLDIR is empty. Bailing out..." >&2
exit 1
fi
mkdir -p "${II2INSTALLDIR}/bin" >/dev/null 2>&1
mkdir -p "${II2INSTALLDIR}/include" >/dev/null 2>&1
mkdir -p "${II2INSTALLDIR}/lib" >/dev/null 2>&1
mkdir -p "${II2INSTALLDIR}/share" >/dev/null 2>&1
mkdir -p "${II2INSTALLDIR}/man/man1" >/dev/null 2>&1
mkdir -p "${II2INSTALLDIR}/man/man4" >/dev/null 2>&1
mkdir -p "${II2INSTALLDIR}/man/man5" >/dev/null 2>&1
if [ ! -w "${II2INSTALLDIR}/bin" -o ! -d "${II2INSTALLDIR}/bin" -o \
! -w "${II2INSTALLDIR}/share" -o ! -d "${II2INSTALLDIR}/share" -o \
! -w "${II2INSTALLDIR}/include" -o ! -d "${II2INSTALLDIR}/include" -o \
! -w "${II2INSTALLDIR}/man/man1" -o ! -d "${II2INSTALLDIR}/man/man1" -o \
! -w "${II2INSTALLDIR}/man/man4" -o ! -d "${II2INSTALLDIR}/man/man4" -o \
! -w "${II2INSTALLDIR}/man/man5" -o ! -d "${II2INSTALLDIR}/man/man5" -o \
! -w "${II2INSTALLDIR}/lib" -o ! -d "${II2INSTALLDIR}/lib" ]
then
echo "### ${PROGNAME}: Cannot write in directories ${II2INSTALLDIR}/{bin,lib,include,man/man1,man/man4,man/man5,share}. Bailing out..." >&2
exit 1
fi
if [ ! -e "${II2INSTALLDIR}/bin"/freetype-config ]
then
echo "### ${PROGNAME}: Freetype2 is missing. Install Freetype first. Bailing out..." >&2
if [ ${II2BUILDSTAMP} -ge 1038004295 ]
then
ANSWER=`"${II2RESOURCES}/doalerter" -s "${II2DOSERVER}" -V -t Alert -T "Component Missing" -I "Freetype2 is missing, without that software, ImageMagick will not work. Do you want to try to open the Freetype i-package?" -1 "Yes" -3 "No"`
if [ "${ANSWER}" == "Yes" ]
then
"${II2RESOURCES}/doalerter" -s "${II2DOSERVER}" -t Open -u "http://tug.org/i-packages/freetype2.ii2"
fi
else
echo "### ${PROGNAME}: A newer version of i-Installer would have opened the Freetype package for you..." >&2
fi
exit 1
fi
echo "### ${PROGNAME}: Finished."
exit 0